<div id="grid"></div>
<script>
// The dataSource is initialized as a stand-alone widget that can be bound to the Grid.
var dataSource = new kendo.data.DataSource({
transport: {
read: {
// The remote endpoint from which the data is retrieved.
url: "https://demos.telerik.com/kendo-ui/service/products",
dataType: "jsonp"
}
},
pageSize: 10
});
$("#grid").kendoGrid({
// The dataSource configuration is set to an existing DataSource instance.
dataSource: dataSource,
pageable: true
});
</script>
The breakpoint is added at the last line (326079) of the file.
The breakpoint is added at the desired line (e.g., 3715).
Describe the bug
The k-toolbar-solid class is not rendered in the Grid's toolbar. As a result, the theme background color is not applied to the toolbar.
To reproduce
Steps to reproduce the behavior:
Expected behavior
The k-toolbar-solid class should be applied to the toolbar's div element, as in the Kendo UI for jQuery Grid and the Core Grid.
The workaround is to add the class with jQuery, for example in the DataBound event of the Grid:
<script>
function onGridDataBound(e) {
$(e.sender.wrapper).find(".k-toolbar").addClass("k-toolbar-solid");
}
</script>
Affected browsers (please remove the unneeded items)
Product Version
2024.4.1112
The jQuery dependency is defined as follows in the package .nuspec file:
<dependency id="jQuery" version="(, 3.7.1]" />
This causes the following warning to appear when installing the package in a project:
Telerik.UI.for.AspNet.Mvc5 2024.3.1015 does not provide an inclusive lower bound for dependency jQuery (<= 3.7.1). jQuery 1.4.1 was resolved instead.
The affected packages are:
Telerik.UI.for.AspNet.Mvc5
Telerik.UI.for.AspNet.Mvc5.Trial
Install the Telerik.UI.for.AspNet.Mvc5 package in a project and check the warnings list.
Warning:
Telerik.UI.for.AspNet.Mvc5 2024.3.1015 does not provide an inclusive lower bound for dependency jQuery (<= 3.7.1). jQuery 1.4.1 was resolved instead.
Add a lower bound (https://learn.microsoft.com/en-us/nuget/reference/errors-and-warnings/nu1604) to resolve the warning about the jQuery dependency.
Bundling the Kendo js files in an ASP.NET MVC application throws a NullReferenceException error. Reproduced with versions 2024.1.319 and 2024.2.514. The bundling works without exceptions in version 2023.3.1114.
bundles.Add(new ScriptBundle("~/bundles/kendo").Include(
"~/Scripts/kendo/2024.2.514/kendo.web.min.js",
"~/Scripts/kendo/2024.2.514/kendo.aspnetmvc.min.js"
));
Instead of kendo.web.min.js you can use kendo.all.min.js with the same result.
@Scripts.Render("~/bundles/kendo")
Exception Details: System.NullReferenceException: Object reference not set to an instance of an object.
Workaround:
Use bundles.Add(new Bundle("~/bundles/kendo") instead of bundles.Add(new ScriptBundle("~/bundles/kendo")
No exception is thrown when bundling the Kendo script files.
I'm trying to modify the filter ui with a custom function that i passed to the kendo UI fuction.
here's my code :
@using Alstom_penali.Models.database;
@{
ViewBag.Title = "Tutte le penali";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<div class="container-fluid">
<div class="row">
<h3>@ViewBag.Title</h3>
@*sotto permessi*@
@if (ViewBag.CanUploadFilePenali == string.Empty)
{
@(Html.Kendo().Upload()
.Name("up_supplychain")
.Messages(y => y.Select("Upload excel penali").UploadSelectedFiles("Avvia"))
.HtmlAttributes(new { accept = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet,application/vnd.ms-excel" })
.Multiple(false)
.ShowFileList(false)
.Events(e => e.Success("onUploadSuccess"))
.Async(a => a
.Save("SuppChainExcelRead", "Summary", new { operation = "save" })
.Remove("SuppChainExcelRead", "Summary", new { operation = "remove" })
.AutoUpload(false)
)
)
<br />
}
@if (ViewBag.CanViewSummaryAll == string.Empty)
{
@(Html.Kendo().Grid<PenaliModel>
()
.Name("grid")
.Events(e => e.ExcelExport("excelExport"))
.Columns(
columns =>
{
columns.Bound(c => c.PO_Number).Title("PO Number").Width(125).Locked(true);
columns.Bound(c => c.Pos).Width(100).Locked(true);
columns.Bound(c => c.ddl_stato).Title("STATO").Width(180).Filterable(false).Sortable(false).Locked(true).ClientTemplate("#=ddl_stato.Text#").EditorTemplateName("DropDownEditor");
columns.Bound(c => c.ddl_Responsabilita).Title("Responsabilità").Width(200).Filterable(false).Sortable(false).Locked(false).ClientTemplate("#=descriptionTemplate(data) #").EditorTemplateName("DropDownEditorResponsabilita");
columns.Bound(c => c.isConQ).Title("Tipo").Width(125)
.Filterable(filter=> filter.UI("isConQRadioFilter"))
.Sortable(false)
.Locked(false)
.ClientTemplate("#=typeTemplate(data) #");
columns.Command(command => { command.Edit().Text("Modifica"); }).Width(100);
columns.Bound(c => c.Progressivo_Conferma).Width(150).Title("CONQ Report");
columns.Bound(c => c.DataInvioConferma).Title("Data Invio CONQ").Width(200).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.NCR).Width(100);
columns.Bound(c => c.SupplierName).Title("Supplier Name").Width(200).Format("{0:n2}");
columns.Bound(c => c.ProgettoSito).Title("MBTO").Width(150);
columns.Bound(c => c.ImportoDaAddebitare).Title("Importo").Width(100).Format("{0:n2}");
columns.Bound(c => c.Importo_Negoziato).Title("Importo Negoziato").Width(150).Format("{0:n2}");
columns.Bound(c => c.Quality_Owner).Title("Quality Owner").Width(200);
columns.Bound(c => c.Progressivo_Avviso).Width(150).Title("ID_CLAIM");
columns.Bound(c => c.DataInvioAvviso).Title("Data Invio CLAIM ").Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.DataInvioPenale).Title("Data Invio penale").Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.NotaDebitoNr).Title("Nota debito").Width(125);
columns.Bound(c => c.DataInvioStorno).Title("Data Invio storno").Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.NotaCreditoNr).Title("Nota credito").Width(125);
columns.Bound(c => c.Id);
columns.Bound(c => c.WBS).Width(200);
columns.Bound(c => c.Progressivo).Width(150);
columns.Bound(c => c.YearMonth).Width(150);
columns.Bound(c => c.Material).Width(200);
columns.Bound(c => c.Description).Width(300).ClientTemplate("<span class='ellipsis'>#:Description#</span>");
columns.Bound(c => c.Del_Note_Nr).Width(150);
columns.Bound(c => c.EmailProcurement).Title("Material planner").Width(200);
columns.Bound(c => c.TotalOrderQty).Width(150);
columns.Bound(c => c.ReceivedQtyInDelay).Width(150);
columns.Bound(c => c.OrderValue).Width(150).Format("{0:n2}");
columns.Bound(c => c.Stat_Del_Date).Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.Del_Date).Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.WeeksOfDelay).Width(150);
columns.Bound(c => c.ReceivedValue).Width(150);
columns.Bound(c => c.DataCreazione).Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Bound(c => c.DataModifica).Width(150).Format("{0:dd/MM/yyyy HH:mm}");
columns.Command(command => { command.Destroy().Text("Elimina"); }).Width(100);
})
.HtmlAttributes(new { style = "height: 500px;" })
.ToolBar(tools => tools.Excel().Text("Esporta excel"))
.Excel(excel => excel.AllPages(true).FileName("Penali.xlsx"))
.Editable(editable =>
{
editable.Mode(GridEditMode.InLine);
editable.ConfirmDelete("Sei sicuro?");
})
.Scrollable()
.Groupable()
.Filterable(filterable => filterable
.Operators(operators => operators
.ForString(str => str.Clear()
.IsEqualTo("E' uguale a").StartsWith("Inizia con").EndsWith("Finisce con")
.Contains("Contiene").DoesNotContain("Non contiene"))
.ForDate(dt => dt.Clear()
.IsGreaterThanOrEqualTo("Dopo o uguale al").IsLessThanOrEqualTo("Prima o uguale del"))
))
.Sortable()
.Selectable()
.Pageable(pageable => pageable
.Refresh(true)
.PageSizes(true)
)
.DataSource(dataSource => dataSource
.Ajax()
.Model(model =>
{
model.Id(p => p.Id);
model.Field(p => p.DataInvioAvviso).Editable(false);
model.Field(p => p.DataInvioPenale).Editable(false);
model.Field(p => p.DataInvioStorno).Editable(false);
model.Field(p => p.DataCreazione).Editable(false);
model.Field(p => p.DataModifica).Editable(false);
model.Field(p => p.Del_Date).Editable(false);
model.Field(p => p.Del_Note_Nr).Editable(false);
model.Field(p => p.Description).Editable(false);
model.Field(p => p.Det_Selected).Editable(false);
model.Field(p => p.EmailProcurement).Editable(false);
model.Field(p => p.ImportoDaAddebitare).Editable(false);
model.Field(p => p.Importo_Negoziato).Editable(false);
model.Field(p => p.Material).Editable(false);
model.Field(p => p.NotaCreditoNr).Editable(false);
model.Field(p => p.NotaDebitoNr).Editable(false);
model.Field(p => p.OrderValue).Editable(false);
model.Field(p => p.PO_Number).Editable(false);
model.Field(p => p.Pos).Editable(false);
model.Field(p => p.ProgettoSito).Editable(false);
model.Field(p => p.Progressivo).Editable(false);
model.Field(p => p.ReceivedQtyInDelay).Editable(false);
model.Field(p => p.ReceivedValue).Editable(false);
model.Field(p => p.Stat_Del_Date).Editable(false);
model.Field(p => p.SupplierName).Editable(false);
model.Field(p => p.TotalOrderQty).Editable(false);
model.Field(p => p.WBS).Editable(false);
model.Field(p => p.WeeksOfDelay).Editable(false);
model.Field(p => p.YearMonth).Editable(false);
model.Field(p => p.NCR).Editable(false);
model.Field(p => p.Progressivo_Avviso).Editable(false);
model.Field(p => p.Progressivo_Conferma).Editable(false);
model.Field(p => p.Quality_Owner).Editable(false);
model.Field(p => p.ddl_stato).DefaultValue(new SelectListItem() { }); //unico editabile
model.Field(p => p.ddl_Responsabilita).DefaultValue(new SelectListItem() { });
model.Field(p => p.isConQ).Editable(false);
model.Field(p => p.DataInvioConferma).Editable(false);
})
.Events(events =>
{
events.RequestEnd("onRequestEnd");
events.Error("onGridError");
})
.Read(read => read.Action("Penali_List", "Summary"))
.Update(update => update.Action("Penali_Update", "Summary"))
.Destroy(update => update.Action("Penali_Destroy", "Summary"))
.PageSize(10)
)
)
}
else
{
<p style="font-size:16px;color:red">@ViewBag.CanViewSummaryAll</p>
}
</div>
</div>
<div id="Pop_up" style="display:none">
<p>Seleziona di chi sarà la responsabilità di questa penale</p>
</div>
@section scripts {
<script type="text/javascript">
function isConQRadioFilter(element) {
// Create radio buttons for filtering boolean values
var html = `
<label>
<input type="radio" name="isConQFilter" value="true" /> CONQ
</label>
<label>
<input type="radio" name="isConQFilter" value="false" /> Ritardo
</label>
`;
// Append the radio button HTML to the filter container
element.append(html);
// Trigger filtering when a radio button is clicked
element.find("input[type=radio]").change(function () {
var filterValue = $(this).val();
var grid = $("#grid").data("kendoGrid");
grid.dataSource.filter({
field: "isConQ",
operator: "eq",
value: filterValue === "true" // Convert the value to a boolean
});
});
}
function onEdit(e) {
}
function excelExport(e) {
var sheet = e.workbook.sheets[0];
var template2 = kendo.template(e.sender.columns[2].template);
var template3 = kendo.template(e.sender.columns[3].template);
var data = e.data;
for (var i = 0; i < data.length; i++) {
sheet.rows[i + 1].cells[2].value = template2(data[i]);
sheet.rows[i + 1].cells[3].value = template3(data[i]);
if (data[i].isConQ) {
sheet.rows[i + 1].cells[4].value = "CONQ";
} else {
sheet.rows[i + 1].cells[4].value = "Ritardo";
}
}
}
function descriptionTemplate(data) {
if ((data.ddl_stato.Text == "INLAVORAZIONE" || data.ddl_stato.Text == "NEGOZIATA") && data.ddl_Responsabilita.Text != null) {
return data.ddl_Responsabilita.Text;
}
else {
return "";
}
}
function typeTemplate(data) {
var html;
if (data.isConQ) {
html = kendo.format(
"<a class=\"k-button\" href='" + '@Url.Action("Create_Penale_CONQ", "Summary")' + "/" + data.Id + " '>CONQ</a> ",
);
} else {
html = kendo.format(
"Ritardo ",
);
}
return html;
}
//(function () {
// isConQFilter("ciao");
//})();
function isConQFilter(element)
{
console.log("isConQFilter called");
element.kendoDropDownList({
dataSource: [
{ text: "CONQ", value: true },
{ text: "Ritardo", value: false }
],
dataTextField: "text",
dataValueField: "value",
optionLabel: "-- Seleziona Tipo --",
valuePrimitive: true
});
}
//function isConQFilter(element) {
// debugger;
// console.log("isConQFilter called");
//}
</script>
}
<style>
.ellipsis {
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-line-clamp: 2;
-webkit-box-orient: vertical;
}
.k-grid-header .k-header {
height: 20px;
padding: 0;
}
.k-grid tbody tr {
line-height: 14px;
}
.k-grid tbody td {
padding: 1px;
}
</style>
the coulm i'm talking about is the "isConq" one, i would like to show "Conq" or "Ritardo" instead of "è vero" or "è falso".
Following the doc, i'm calling the "isConQFilter" js function, but it is never triggered by the kendo.
Where am i wronged?
If you need something else, please, tell me.
I'm using kendo mvc grid version 2016.
Regression in R1 2023.
columns.Bound(p => p.OrderDate).HtmlAttributes(new { title = "Order Date: #=kendo.toString(OrderDate, 'dd-MM-yyyy')# " });
kendo.toString is not executed and as a result the date is not formatted. The exact value of the title attribute, as shown above is rendered as title of the cell.
The logic is executed and the OrderDate value is rendered in the title with the specified format.
After i upgrade my ASP.NET MVC project to Telrik UI version 2024.2.514, many component in my web app in smaller than before upgrade. Some form are broken and unable to use. Do you have any idea causes this issue?
I'm looking forward to your answser. Thanks for your support.
Hi Team,
1. Date column filter value shown full date instead of dd/MM/yyyy format. If I change the format filter not working.
Sample screenshot:
2. When I click on grid or some other area on the page selected filter text value like('Last month', 'Last week', 'Today', 'Yesterday') from custom date filter dropdown
Grid:
@(Html.Kendo().Grid<InteractiveReports.Model.ViewModel.UserReportResult>()
.Name("userreport")
.HtmlAttributes(new { @class = "reportgrid" })
//.ToolBar(toolBar => toolBar.Template(
//"<a id='exportpdfdata' class='k-button k-button-icontext k-grid' href='#'><span class='k-icon k-i-pdf'></span>Export to PDF</a>" +
//"<a class='k-button k-button-icontext k-grid-excel' href='#'><span class='k-icon k-i-excel'></span>Export to Excel</a>" +
//"<a id='exportcsvdata' class='k-button k-button-icontext k-grid-csv' href='#'><span class='k-icon k-i-csv'></span>Export to CSV</a>"
//))
.Excel(excel => excel
.AllPages(true)
.FileName("User Report.xlsx")
.Filterable(true)
)
.Columns(columns =>
{
columns.Bound(c => c.Team).Title("Team Name").Filterable(ftb => ftb.Multi(true).Cell(cell => cell.ShowOperators(false).Template("TeamDropDownList")))
.Locked(true)
.ClientTemplate("<a class = 'link-button' title='Team Report' href='\\#' onclick=" + " onteamClick('#=escape(Team)#');>" + "#= Team != null && Team != '(Blanks)' ? Team : '' #" + "</a>")
.Width(200);
//columns.Bound(c => c.Team_type).Title("Team type").Filterable(ftb => ftb.Multi(true)).Width(200);
columns.Bound(c => c.Team_type).Title("Team type").Filterable(ftb => ftb.Multi(true).Cell(cell => cell.ShowOperators(false).Template("Team_typeDropDownList")))
.Width(200).ClientTemplate("#= (Team_type == '(Blanks)') ? '' : Team_type #");
columns.Bound(c => c.Username).Title("Username")
.Width(200).ClientTemplate("#= (Username == '(Blanks)') ? '' : Username #");
columns.Bound(c => c.Title).Title("Title").Width(200).ClientTemplate("#= (Title == '(Blanks)')? '' : Title #");
columns.Bound(c => c.First_name).Title("First Name").Width(200).ClientTemplate("#= (First_name == '(Blanks)') ? '' : First_name #");
columns.Bound(c => c.Last_name).Title("Last Name").Width(200).ClientTemplate("#= (Last_name == '(Blanks)') ? '' : Last_name #");
columns.Bound(c => c.Email_address).Title("Email Address")
.Width(200).ClientTemplate("#= (Email_address == '(Blanks)') ? '' : Email_address #");
columns.Bound(c => c.Company).Title("Company").Filterable(ftb => ftb.Multi(true).Cell(cell => cell.ShowOperators(false).Template("CompanyDropDownList")))
.Width(200).ClientTemplate("#= (Company == '(Blanks)') ? '' : Company #");
columns.Bound(c => c.User_status).Title("User Status").Filterable(ftb => ftb.Multi(true).Cell(cell => cell.ShowOperators(false).Template("User_statusDropDownList")))
.Width(200).ClientTemplate("#= (User_status == '(Blanks)') ? '' : User_status #");
columns.Bound(c => c.Total_time_logged_in).Title("Total Time Logged In").Width(200)
.ClientTemplate("#= (Total_time_logged_in == '(Blanks)') ? '' : Total_time_logged_in #");
columns.Bound(c => c.Last_login_date).Title("Last Login Date").Width(200)
//.ClientTemplate("#= Last_login_date != null ? Last_login_date : '' #")
.ClientTemplate("#= Last_login_date != null ? kendo.toString(Last_login_date, 'dd/MM/yyyy') : '' #")
.Format("{0:dd/MM/yyyy}")
//.Filterable(ftb => ftb.Cell(cell => cell.Template("datePickerFilter")));
.Filterable(f => f.Cell(c => c.ShowOperators(false).Template("dateFilterCustomization")));
columns.Bound(c => c.Last_login_time).Title("Last Login Time").Width(200)
.ClientTemplate("#= (Last_login_time == '(Blanks)') ? '' : Last_login_time #");
//.Filterable(f => f.Cell(c => c.ShowOperators(false).Template("timeFilterCustomization")));
columns.Bound(c => c.No_of_Index_Actions).Title("No. of Index Actions").Width(200)
.ClientTemplate("<a class = 'link-button' title='Index Report' href='\\#' onclick=" + " onidxActionClick('#=escape(UserId)#');>" + "#= No_of_Index_Actions != null ? No_of_Index_Actions : '' #" + "</a>")
.Filterable(ftb => ftb
.Cell(cell => cell
.Operator("eq")
.ShowOperators(false)
.Template("integerFilter_nodecimal")
)); })
.NoRecords(n => n.Template("NO DATA TO DISPLAY"))
.Events(events => events.Filter("onFiltering"))
.Resizable(resize => resize.Columns(true))
.Reorderable(reorder => reorder.Columns(true))
.ColumnMenu()
.Sortable()
.Scrollable()
.Filterable(ftb => ftb.Mode(GridFilterMode.Row))
.Events(events => events.Filter("onFilter"))
.Pageable(pageable => pageable
.Refresh(true)
.PageSizes(true)
.PageSizes(new[] { 50, 100, 150 })
)
.DataSource(dataSource => dataSource
.Ajax()
.ServerOperation(false)
.Read(read => read.Action("GetUserReportData", "UserReport").Data("passParameter"))
.PageSize(50).Events(x => x.Error("onGridError"))
)
)
Customized Dropdown filter for date column:
var field = {};
var filter = true;
var dateformate = "DD/MM/YYYY";
const formatter = new Intl.DateTimeFormat(['ban', 'id'], { day: '2-digit', month: '2-digit', year: 'numeric' });
function dateFilterCustomization(args) {
var date = new Date();
var yesterday = new Date(date);
yesterday.setDate(date.getDate() - 1);
args.element.kendoComboBox({
dataSource: [
{ text: "Today", value: "1" },
{ text: "Yesterday", value: "2" },
{ text: "This Week", value: "3" },
{ text: "Last Week", value: "4" },
{ text: "Last Month", value: "5" },
{ text: "Since Live", value: "6" },
{ text: "Custom Date range", value: "7" },
],
placeholder: "Select",
dataTextField: "text",
dataValueField: "value",
dataTypeField: "",
valuePrimitive: true,
value: 7,
open: function (e) {
var grid = $('.reportgrid').data("kendoGrid");
var colname = e.sender.element[0].title;
field = getColName(grid.columns, colname);
if (grid.dataSource.filter() != undefined) {
var filters = checkForExistingDates(grid);
if (filters != undefined && filters.length > 0) {
var result = jQuery.grep(filters, function (item) { return item.operator == "gte" })
if (result != undefined && result.length > 0) {
$("#startDate").val(formatter.format(result[0].value));
}
else {
$("#startDate").val('');
}
result = jQuery.grep(filters, function (item) { return item.operator == "lte" })
if (result != undefined && result.length > 0) {
$("#endDate").val(formatter.format(result[0].value));
} else {
$("#endDate").val('');
}
}
else {
$("#startDate").val('');
$("#endDate").val('');
}
} else {
$("#startDate").val('');
$("#endDate").val('');
}
},
select: function (e) {
e.preventDefault();
var dataItem = e.dataItem;
var grid = $('.reportgrid').data("kendoGrid");
var colname = e.sender.element[0].title;
field = getColName(grid.columns, colname);
var vm = this;
var text = dataItem.text;
flag = parseInt(dataItem.value);
if (flag == 1) {
filterSingleDate(date);
} else if (flag == 2) {
filterSingleDate(yesterday);
} else if (flag == 3) {
getThisWeekData();
} else if (flag == 4) {
getPastWeekData();
} else if (flag == 5) {
getPastMonthData();
} else if (flag == 6) {
removeFilter(grid);
}
else if (flag == 7) {
$(".daterangemodel").modal('toggle');
}
vm.text(text);
}
});
}
function startOfLastWeek() {
var today = moment();
var daystoLastMonday = 0 - (1 - today.isoWeekday()) + 7;
var lastMonday = today.subtract('days', daystoLastMonday);
return lastMonday;
}
function EndOfLastWeek() {
var lastMonday = startOfLastWeek();
var lastSunday = lastMonday.add('days', 6);
return lastSunday;
}
function getPastWeekData() {
var startDate = startOfLastWeek();
var endDate = EndOfLastWeek();
filterData(startDate, endDate, field);
}
function getThisWeekData() {
var date = new Date();
var startDate = startOfThisWeek(date);
var endDate = endOfThisWeek(date);
filterData(startDate, endDate, field);
}
Script:
function onFilter(e) {
if (e.filter && e.filter.filters[0].field == "Last_login_date" || e.filter && e.filter.filters[0].field == "Creation_date" || e.filter && e.filter.filters[0].field == "Invitation_date" || e.filter && e.filter.filters[0].field == "Registration_date") {
Model:
public class UserReportResult
{
public string Team { get; set; }
public string Team_type { get; set; }
public string Username { get; set; }
public string Title { get; set; }
public string First_name { get; set; }
public string Last_name { get; set; }
public DateTime ? Last_login_date { get; set; }
}
Can any admin tell me what is wrong??
In a Selectable grid, when only one column is shown in the grid, and the model's Id field is specified, an error occurs on selection.
If you include more than one column (must be more than one visible) it works correctly.
If the model's Id is not specified, it works correctly even with only one column visible.
@(Html.Kendo().Grid<TelerikMvcApp1.Models.OrderViewModel>()
.Name("grid1")
.Columns(columns => {
columns.Bound(p => p.ShipName);
columns.Bound(p => p.ShipCity).Hidden(true);
})
.Pageable()
.Sortable()
.Scrollable()
.Filterable()
.Selectable(s => s.Mode(GridSelectionMode.Single))
.HtmlAttributes(new { style = "height:430px;" })
.DataSource(dataSource => dataSource
.Ajax()
.PageSize(20)
.Read(read => read.Action("Orders_Read", "Grid"))
.Model(m => m.Id("OrderID"))
)
)
Dear team,
Recently I've updated the kendo UI.
currently I'm using the this version.
Here I wrote the code for testing.
// cshtml code
@(Html.Kendo().Grid<Alliant.Controllers.ProductViewModel>()
.Name("mixedSort")
.Columns(columns => {
columns.Bound(o => o.ProductName).Width(300);
columns.Bound(p => p.UnitPrice).Width(300);
columns.Bound(p => p.UnitsInStock).Width(300);
columns.Bound(p => p.Discontinued).Width(300);
columns.Bound(p => p.Group).Width(300);
})
.Pageable(pageable => pageable.ButtonCount(5))
.Sortable(sortable => sortable
.AllowUnsort(true)
.SortMode(GridSortMode.Mixed)
.ShowIndexes(true))
.DataSource(dataSource => dataSource
.Ajax()
.PageSize(5)
.Read(read => read.Action("Sorting_Orders_Read", "Testing"))
)
)
// Controller code
public ActionResult Sorting_Orders_Read([DataSourceRequest] DataSourceRequest request)
{
List<ProductViewModel> lstData = new List<ProductViewModel>()
{
new ProductViewModel(){Group="A",ProductName="A1",UnitPrice=10,Discontinued=1,UnitsInStock=50},
new ProductViewModel(){Group="A",ProductName="B1",UnitPrice=20,Discontinued=2,UnitsInStock=60},
new ProductViewModel(){Group="B",ProductName="C1",UnitPrice=30,Discontinued=3,UnitsInStock=70},
new ProductViewModel(){Group="C",ProductName="D1",UnitPrice=40,Discontinued=4,UnitsInStock=80},
new ProductViewModel(){Group="C",ProductName="E1",UnitPrice=50,Discontinued=5,UnitsInStock=90},
};
return Json(lstData.ToDataSourceResult(request), JsonRequestBehavior.AllowGet);
}
// Modelpublic class ProductViewModel
{
public string ProductName { get; set; }
public decimal UnitPrice { get; set; }
public int UnitsInStock { get; set; }
public decimal Discontinued { get; set; }
public string Group { get; set; }
}
Could you please help me to figure out the issue?
I also checked the jquery code.
// Jquery code render by kendo
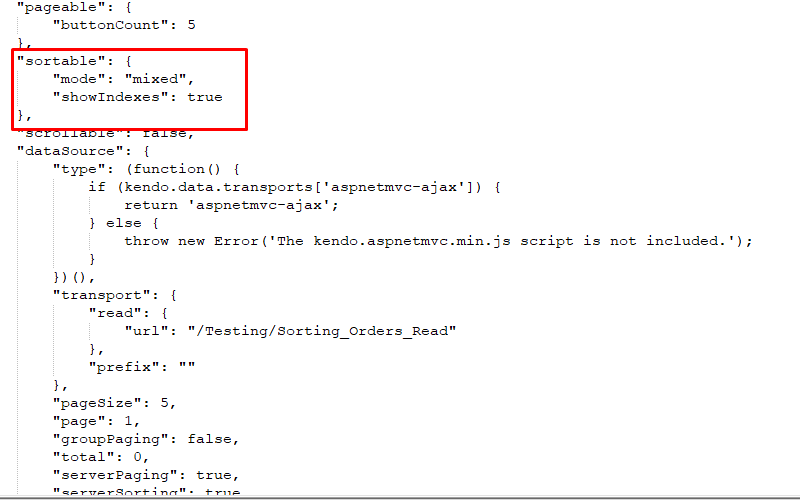
I have a cshtml page that uses Kendo UI ASP.NET MVC that does the following:
The view contains one or more grid widgets (the number depends on how many result types were requested) and each grid is set up to export Excel.
The problem is that when window.open() is used to open a new tab, the browser history state is null and the window.location.href is empty with the browser location showing "about:blank". For Chrome and Firefox, this does not cause any issues when exporting excel for the grid. However, when using Edge with this situation, when the Export to Excel grid toolbar button is clicked and the onExportExcel event is fired, the browser Open or Save dialog prompt is displayed but the active tab (the one that contained the grid) closes. This behaviour is very undesireable. The ProxyUrl grid excel option does not fire since Edge supports javascript file saving.
A workaround for this situation is to forcibly set the new tab window object location href by using the window.history.pushUpdate function. A code snippet is included below.
let dataModel = {Id = 123571113, Name="jason bourne"}; let jsonModel = JSON.stringify(dataModel); let curDate = new Date(); let targetUrl = '@Url.Action("Reports", "Report", new { @area = "Reports" })'; let targetWindowName = "something meaningful" + " " + curDate.toISOString();//add datetime stamp to avoid issue where you cannot open a window with the same name as the current window. let html = "some html content to provide a temporary message to your audience"; let targetWindow = window.open(targetUrl, targetWindowName); if (targetWindow !== null && targetWindow !== undefined) { targetWindow.document.write(html); targetWindow.document.close(); // to finish loading the page targetWindow.document.title = targetWindowName; //attempt to forcibly update the URL in the history and target window location to fix problem with grid export to excel on Edge browser let targetWindowHistoryHref = window.location.href; if (oModel.BuildingDesigns !== null && oModel.BuildingDesigns !== undefined && oModel.BuildingDesigns.length > 0) { targetWindowHistoryHref += "?oBuildingDesignId=" + oModel.BuildingDesigns[0].ObfuscatedBuildingDesignId + "&BuildingDesignName=" + oModel.BuildingDesigns[0].BuildingDesignName; } else { targetWindowHistoryHref += "?" + oModel.Target; } targetWindow.history.pushState(null, null, targetWindowHistoryHref); } $.when( $.ajax({ type: "POST", dataType: "html", // this is the data type expected to be returned from the controller method contentType: "application/json", // this is the content type expected by the controller method url: targetUrl, data: jsonModel, beforeSend: function() { console.log(".... submitting report request"); athena.loader.loading("submitting report request"); }, success: function(response) { athena.loader.stopLoading(); if (debugLevel > 2) { console.log(".... response = " + response + " : ", response); } //attempt to populate the target browser tab with the response try { console.log(".... attempting to open a browser tab and populate it with the HTML response object"); console.log(".... targetWindow = " + targetWindow); //will trigger popup blockers :: targetWindow = window.open("", oModel.Target); if (targetWindow !== null) { if (response === null || response === undefined) { targetWindow.document.body.innerHTML = ''; } else { //completely replace the existing document (not just the innerhtml) targetWindow.document.open(); targetWindow.document.write(response); targetWindow.document.close(); targetWindow.document.title = targetWindowName; } } } catch (ex) { // do nothing, just catch when the open fails. console.log("error: " + ex.message); } }, error: function(jqXhr, textStatus, errorThrown) { console.log('.... error :: ajax status = ' + textStatus + ' :: errorThrown = ' + errorThrown); console.log('....-- jqXhr.responseText :: \n' + jqXhr.responseText); } }) ).done( function() { console.log(".... report request has completed"); targetWindow.focus(); } );
Hi,
We are using globalization with UI for ASP.NET MVC to translate control to French (fr-CA). However, while most messages are correctly displayed in French, there are some that are still displayed in English. For example, if we use the Grid control with filter on a 'string' column, the dropdown for the operators shows both French ("Commence par") and English ("Is empty") operators:
Looking at the source code, we can see that in ressource files "Messages.fr-FR.resx" and "Messages.fr-CA.resx", there are some messages that are still in English (for example, Filter_StringIsEmpty).
Note that corresponding text seems to be correctly translated in jQuery messages file (for example, "kendo.messages.fr-CA.js"), so it might only be a matter of applying the same translation to .resx files.
Note also that the same problem seems to be present in the source code of the latest version (2020.1.114).
Thanks!
After using setOptions() the HTML structure of Grids column header is different.
In the following demo:
https://demos.telerik.com/aspnet-mvc/grid/persist-state
When the button is clicked, setOptions() called for the Grid and the structure of the column headers is now different.
When setOpitons() is called with no options that may affect the headers the HTML structure of the column headers should be the same.
My web page displays grids on multiple pages, and each grid has a pager with a page size control.
In recent updates the styling broke in all but one page.
In the case where I was creating the grid using Kendo UI for JQuery, it worked fine.
In the instances when I create it using MVC, there are styling errors. (See in the image how the number "10" is truncated.)
After much drilling into the HTML, I found the difference in how the Razor library creates the HTML elements, as opposed to how the JQuery creates the HTML elements.
JQuery generates the following element structure. Note that the k-pager-sizes element comes after the k-pager-numbers-wrap element
<div class="k-pager k-grid-pager k-pager-md"> <!-- The pager bar -->
<div class="k-pager-numbers-wrap">
<!-- ... -->
</div>
<span class="k-pager-sizes k-label">
<!-- The page-size picker goes here -->
</span>
</div>
In contrast, the Kendo Razor libraries generates the following element structure, where the k-pager-sizes element falls inside the k-pager-numbers-wrap element:
<div class="k-pager k-grid-pager k-pager-md"> <!-- The pager bar -->
<div class="k-pager-numbers-wrap">
<!-- ... -->
<span class="k-pager-sizes k-label">
<!-- The page-size picker goes here -->
</span>
</div>
</div>
This breaks the styling. (I am using the bootstrap kendo theme) There is styling rule that imposes a minimum width on all buttons under .k-pager-numbers-wrap. This makes the drop-down arrow too wide, which causes the page size number to truncate.
@each $size, $size-props in $kendo-pager-sizes {
$_padding-x: k-map-get($size-props, padding-x);
$_padding-y: k-map-get($size-props, padding-y);
$_item-group-spacing: k-map-get($size-props, item-group-spacing);
$_item-min-width: k-map-get($size-props, item-min-width);
$_pager-dropdown-width: k-map-get($size-props, pager-dropdown-width);
.k-pager-#{$size} {
padding-inline: $_padding-x;
padding-block: $_padding-y;
gap: $_item-group-spacing;
.k-pager-numbers-wrap {
.k-button {
min-width: $_item-min-width;
}
My current hack workaround is to add a more specific CSS rule.
@import "@progress/kendo-theme-bootstrap/dist/all.scss";
.k-pager-md .k-pager-numbers-wrap .k-dropdownlist .k-button {
min-width:inherit;
}
Please note the following MVC code:
Bug 1: The Window should have the top set by the Top() method. It does not.
Bug 2: The Window should have the top set by the HtmlAttributes() method. That does not work either.
Bug 3: The scrollable method also does nothing to the window. So this entire line seems to be omitted.
Bug 4: The window is supposed to be centered by the Kendo native code. It isn’t. The recurring editor div element is not shown until after the window centers. So the window generates, centers on the screen, then the recurring DIV is shown which expands the window downwards, then the window is shown. This makes it go off the bottom of the screen. I didn’t need vertical center anyways so I fixed this by setting the top to 40px. Which is when I ran into the above issues. However if I was not doing this, the center() issue would still be there. I fixed this in JavaScript on the event’s edit event.