Use attached to reproduce and for workaround.
My current theme is windows10. In two images I demonstrate the problem.
Controls in RadPanel redraw slowly when changing the Enabled state of the panel. Workaround: private void radButton1_Click(object sender, EventArgs e) { if (this.radPanel1.Enabled) { this.radPanel1.Enabled = false; } else { this.radPanel1.Visible = false; this.radPanel1.Enabled = true; this.radPanel1.Visible = true; } }
The issue reproduces if the panels are collapsed before auto hiding the windows. When the window is later displayed the collapsed panels cannot be expanded. Check the attached video: Workaround: Cache the old bounds. public partial class Form1 : Form { public Form1() { InitializeComponent(); this.radDock1.AutoHideWindowDisplaying += RadDock1_AutoHideWindowDisplaying; this.radDock1.AutoHideWindowDisplayed += RadDock1_AutoHideWindowDisplayed; this.radCollapsiblePanel1.PanelContainer.BackColor = Color.LightGreen; } Rectangle cache; private void RadDock1_AutoHideWindowDisplayed(object sender, DockWindowEventArgs e) { this.radCollapsiblePanel1.OwnerBoundsCache = this.cache; } private void RadDock1_AutoHideWindowDisplaying(object sender, Telerik.WinControls.UI.Docking.AutoHideWindowDisplayingEventArgs e) { this.cache = this.radCollapsiblePanel1.OwnerBoundsCache; } }
To reproduce: 1. Place label on form. 2. Set text alignment property to middle center. 3. Change label property auto-size to false. 4. Resize control (width wise). 5. Text still sits on left side of the label and not the middle of the label. The text is properly aligned at runtime.
To reproduce: add a RadCollapsiblePanel and insert several controls at run time with specifying the location and size of the inner controls. Set the ContentSizingMode property to CollapsiblePanelContentSizingMode.FitToContentHeight | CollapsiblePanelContentSizingMode.FitToContentWidth. When you run the application you will notice that the inner controls are cut off and the panel is not resized according to its content.
Workaround:
Before:
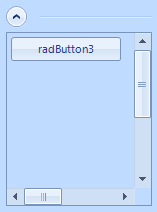
After:
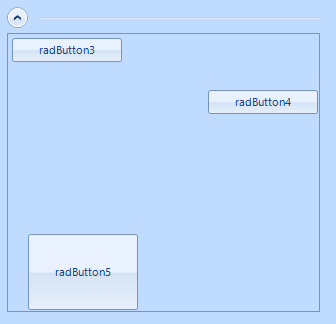
public RadForm2()
{
InitializeComponent();
this.radCollapsiblePanel1.ContentSizingMode = CollapsiblePanelContentSizingMode.None;
}
private void RadForm2_Load(object sender, EventArgs e)
{
SizeF fillElementSize = this.radCollapsiblePanel1.Size;
int maxY = int.MinValue;
int maxX = int.MinValue;
int maxControlWidth = int.MinValue;
int maxControlHeight = int.MinValue;
bool fitToHeight = true;
bool fitToWidth = true;
Padding panelContainerPadding = this.radCollapsiblePanel1.ControlsContainer.PanelContainer.Padding;
foreach (Control control in this.radCollapsiblePanel1.ControlsContainer.PanelContainer.Controls)
{
if (fitToHeight)
{
maxY = Math.Max(maxY, control.Bounds.Location.Y + control.Bounds.Size.Height + control.Margin.Bottom + panelContainerPadding.Bottom +
this.radCollapsiblePanel1.ControlsContainer.VerticalScrollbar.Value);
maxControlWidth = Math.Max(maxControlWidth, control.Width);
}
if (fitToWidth)
{
maxX = Math.Max(maxX, control.Bounds.Location.X + control.Bounds.Size.Width + control.Margin.Right + panelContainerPadding.Right +
this.radCollapsiblePanel1.ControlsContainer.HorizontalScrollbar.Value);
maxControlHeight = Math.Max(maxControlHeight, control.Height);
}
}
if (fitToHeight)
{
bool horizontalScrollbarNeeded = fillElementSize.Width <= maxY + maxControlWidth;
if (horizontalScrollbarNeeded)
{
this.radCollapsiblePanel1.ControlsContainer.HorizontalScrollBarState = ScrollState.AlwaysHide;
}
else
{
this.radCollapsiblePanel1.ControlsContainer.HorizontalScrollBarState = ScrollState.AutoHide;
}
fillElementSize.Height = maxY;
}
if (fitToWidth)
{
bool verticalScrollbarNeeded = fillElementSize.Height <= maxX + maxControlHeight;
if (verticalScrollbarNeeded)
{
this.radCollapsiblePanel1.ControlsContainer.VerticalScrollBarState = ScrollState.AlwaysHide;
}
else
{
this.radCollapsiblePanel1.ControlsContainer.VerticalScrollBarState = ScrollState.AutoHide;
}
fillElementSize.Width = maxX;
}
fillElementSize.Height += this.radCollapsiblePanel1.CollapsiblePanelElement.HeaderElement.Size.Height + 5;
this.radCollapsiblePanel1.MinimumSize = this.radCollapsiblePanel1.MaximumSize = this.radCollapsiblePanel1.Size = fillElementSize.ToSize();
}
How to reproduce: CollapsiblePanelHeaderElement headerElement = this.radCollapsiblePanel1.CollapsiblePanelElement.HeaderElement; RadTextBoxElement txtBox = new RadTextBoxElement(); headerElement.Children.Add(txtBox); Workaround: use RadTextBoxControlElement
When RadPanel is set to AutoSize, it should update its size automatically when changing the visibility of some control inside it. Workaround: public class CustomRadPanel : RadPanel { public override string ThemeClassName { get { return typeof(RadPanel).FullName; } set { base.ThemeClassName = value; } } protected override void OnLayout(LayoutEventArgs e) { base.OnLayout(e); int maxHeight = 0; foreach (Control control in this.Controls) { if (control.Visible) { maxHeight = Math.Max(control.Height, maxHeight); } } this.AutoSize = false; this.Height = maxHeight; } }
To reproduce: Add a RadLabel to a Form. Set the Margin and TextAlignment properties as well as some text: this.radLabel1.AutoSize = true; this.radLabel1.LabelElement.LabelText.Margin = new System.Windows.Forms.Padding(10, 10, 10, 10); this.radLabel1.TextAlignment = System.Drawing.ContentAlignment.MiddleCenter; this.radLabel1.Text = "This text should appear middle-center"; You will see that the text is not aligned at the center of the label. Workaround: Set the Margin to the layout panel instead: this.radLabel1.LabelElement.Children[2].Margin = new Padding(10); Or from the ElementEditor -> LabelElement -> ImageAndTextLayoutPanel
If I add a RadLabel to a RadPanel, and set the panel AutoScroll to true, scrolling the panel will cause lines to appear. The words get clipped.
To reproduce: - Add a scrollable panel wit other controls in a form. - Show the form using the ShowDialog method. - Scroll to the bottom the close and show the form again - You will notice that the scrollbar is not shown and the controls at the top are not visible. Workaround: Protected Overrides Sub OnClosing(e As System.ComponentModel.CancelEventArgs) MyBase.OnClosing(e) RadScrollablePanel1.VerticalScrollbar.Value = 0 End Sub
How to reproduce: this.radScrollablePanel1.PanelElement.BorderThickness = new Padding(0); Workaround: in order to hide the border of the RadScrollablePanel.PanelElement its Visiblity needs to be set to Hidden or Collapsed this.radScrollablePanel1.PanelElement.Border.Visibility = Telerik.WinControls.ElementVisibility.Hidden;
To reproduce: Add a RadScrollablePanel and a button at a location of 500, 1000. Start the app and click the panel(not the button) you will see that you cannot scroll the panel. Workaround: Subscribe to the MouseWheel event of RadScrollablePanel: void RadScrollablePanel_MouseWheel(object sender, MouseEventArgs e) { typeof(Control).GetMethod("OnMouseWheel", System.Reflection.BindingFlags.Instance | System.Reflection.BindingFlags.NonPublic).Invoke(scrollablePanel.PanelContainer, new object[] { e }); }
To reproduce: - Put grid and collapsible panel to a form. - Set the Dock of the grid to fill. - Set the Dock of the panel to top. - Expand the panel, you will notice that the panel is drawn over the grid. Workaround: public RadForm1() { InitializeComponent(); radGridView1.Anchor = AnchorStyles.None; radCollapsiblePanel1.Dock = DockStyle.Top; radCollapsiblePanel1.EnableAnimation = false; radCollapsiblePanel1.Expanded += radCollapsiblePanel1_Expanded; radCollapsiblePanel1.Collapsed += radCollapsiblePanel1_Collapsed; gridSmallsize = new Size(this.Width - 10, this.Height - 190); gridLargeSize = new Size(this.Width - 10, this.Height - 60); } Size gridSmallsize; Size gridLargeSize; void radCollapsiblePanel1_Collapsed(object sender, EventArgs e) { radGridView1.Size = gridLargeSize; radGridView1.Location = new Point(0, 30); } void radCollapsiblePanel1_Expanded(object sender, EventArgs e) { radGridView1.Size = gridSmallsize; radGridView1.Location = new Point(0, 160); }
To reproduce: 1. Add a RadSplitContainer with two split panels. 2. Add a RadCollapsiblePanel to the left split panel and set the RadCollapsiblePanel.Dock property to Fill. 3. Add a RadGridView inside the RadCollapsiblePanel and set the RadGridView.Dock property to Fill. 4. Populate the grid with data. 5. Run the application and move the splitter to increase the RadCollapsiblePanel's size. The RadGridView is resized correctly. 6. Move the splitter to shrink the RadCollapsiblePanel. As a result the grid is not resized correctly. Workaround: Subscribe to the RadSplitContainer.SplitterMoved event and use the following code: this.RadCollapsiblePanel1.EnableAnimation = false; this.RadCollapsiblePanel1.IsExpanded = !this.RadCollapsiblePanel1.IsExpanded; this.RadCollapsiblePanel1.IsExpanded = !this.RadCollapsiblePanel1.IsExpanded; this.RadCollapsiblePanel1.EnableAnimation = true;